

Floating-point remainderįor the float and double operands, the result of x % y for the finite x and y is the value z such that Use the Math.DivRem method to compute both integer division and remainder results. The sign of the non-zero remainder is the same as that of the left-hand operand, as the following example shows: Console.WriteLine(5 % 4) // output: 1Ĭonsole.WriteLine(-5 % -4) // output: -1 Integer remainderįor the operands of integer types, the result of a % b is the value produced by a - (a / b) * b. The remainder operator % computes the remainder after dividing its left-hand operand by its right-hand operand. For more information about conversions between numeric types, see Built-in numeric conversions. You must explicitly convert the float or double operand to the decimal type. If one of the operands is decimal, another operand can be neither float nor double, because neither float nor double is implicitly convertible to decimal. To obtain the quotient of the two operands as a floating-point number, use the float, double, or decimal type: Console.WriteLine(13 / 5.0) // output: 2.6Ĭonsole.WriteLine((double)a / b) // output: 2.6įor the float, double, and decimal types, the result of the / operator is the quotient of the two operands: Console.WriteLine(16.8f / 4.1f) // output: 4.097561Ĭonsole.WriteLine(16.8d / 4.1d) // output: 4.09756097560976Ĭonsole.WriteLine(16.8m / 4.1m) // output: 4.0975609756097560975609756098 Integer divisionįor the operands of integer types, the result of the / operator is of an integer type and equals the quotient of the two operands rounded towards zero: Console.WriteLine(13 / 5) // output: 2Ĭonsole.WriteLine(-13 / 5) // output: -2Ĭonsole.WriteLine(13 / -5) // output: -2Ĭonsole.WriteLine(-13 / -5) // output: 2 The division operator / divides its left-hand operand by its right-hand operand. The unary * operator is the pointer indirection operator. The multiplication operator * computes the product of its operands: Console.WriteLine(5 * 2) // output: 10Ĭonsole.WriteLine(0.5 * 2.5) // output: 1.25Ĭonsole.WriteLine(0.1m * 23.4m) // output: 2.34 The ulong type doesn't support the unary - operator. Console.WriteLine(+4) // output: 4Ĭonsole.WriteLine(b.GetType()) // output: System.Int64Ĭonsole.WriteLine(-double.NaN) // output: NaN The unary - operator computes the numeric negation of its operand.
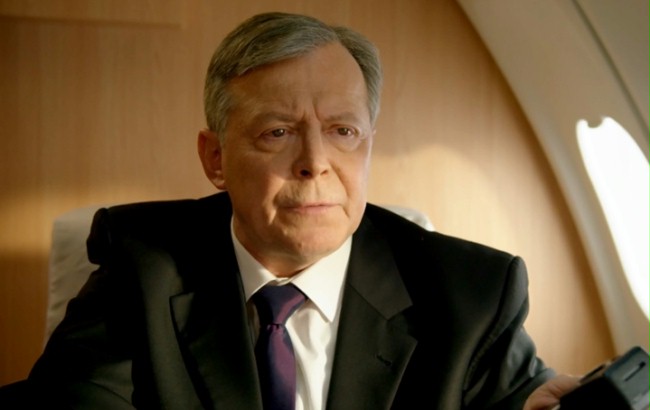
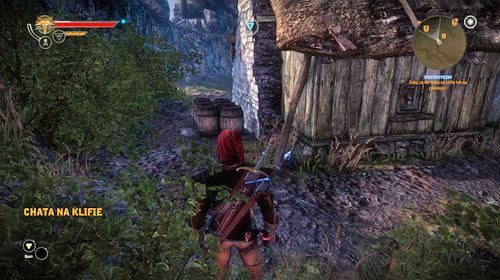
The unary + operator returns the value of its operand. The result of -x is the value of x after the operation, as the following example shows: double a = 1.5 The result of x- is the value of x before the operation, as the following example shows: int i = 3 The decrement operator is supported in two forms: the postfix decrement operator, x-, and the prefix decrement operator, -x. The operand must be a variable, a property access, or an indexer access. The unary decrement operator - decrements its operand by 1. The result of ++x is the value of x after the operation, as the following example shows: double a = 1.5 The result of x++ is the value of x before the operation, as the following example shows: int i = 3 The increment operator is supported in two forms: the postfix increment operator, x++, and the prefix increment operator, ++x. The unary increment operator ++ increments its operand by 1. The ++ and - operators are defined for all integral and floating-point numeric types and the char type. For more information, see the Numeric promotions section of the C# language specification. When operands are of different integral or floating-point types, their values are converted to the closest containing type, if such a type exists. When operands are of other integral types ( sbyte, byte, short, ushort, or char), their values are converted to the int type, which is also the result type of an operation. In the case of integral types, those operators (except the ++ and - operators) are defined for the int, uint, long, and ulong types. Those operators are supported by all integral and floating-point numeric types. Binary * (multiplication), / (division), % (remainder), + (addition), and - (subtraction) operators.Unary ++ (increment), - (decrement), + (plus), and - (minus) operators.The following operators perform arithmetic operations with operands of numeric types:
